异常过滤器
异常过滤器
Nest 带有一个内置的异常层,负责处理应用中所有未处理的异常。当你的应用代码未处理异常时,该层会捕获该异常,然后自动发送适当的用户友好响应。
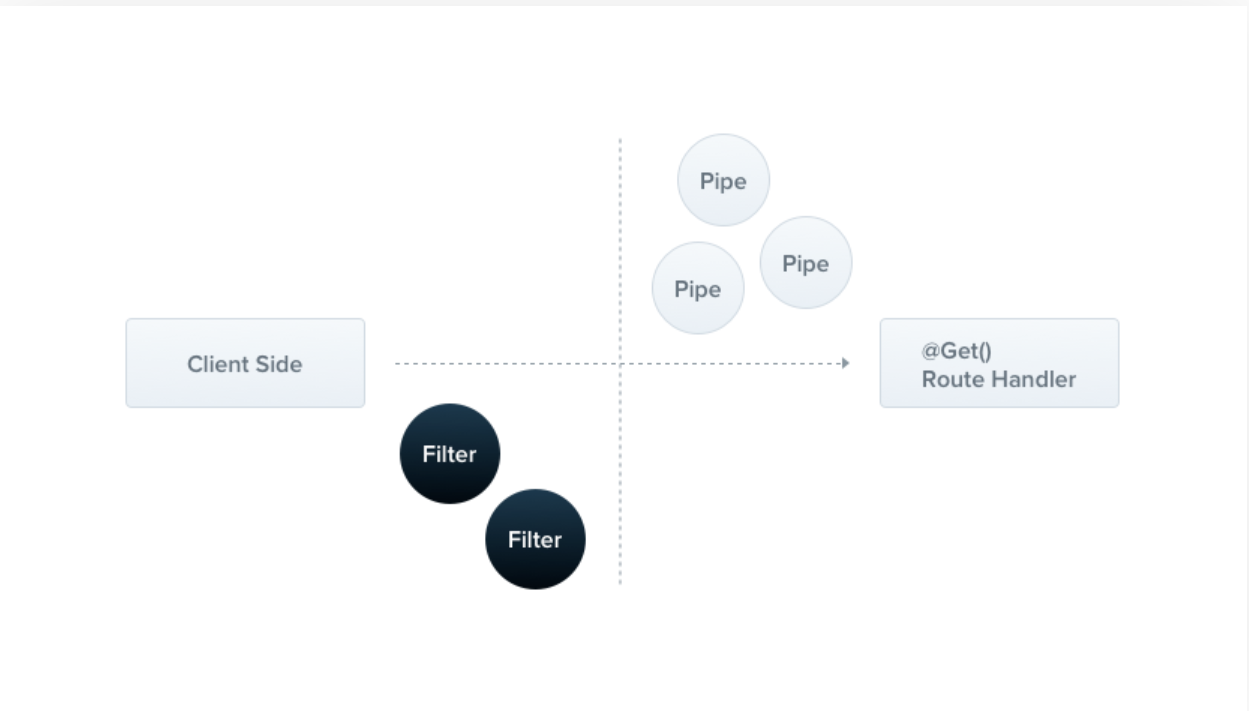
开箱即用,此操作由内置的全局异常过滤器执行,该过滤器处理 HttpException
类型(及其子类)的异常。当异常无法识别时(既不是 HttpException
也不是继承自 HttpException
的类),内置异常过滤器会生成以下默认 JSON
响应
{
"statusCode": 500,
"message": "Internal server error"
}
注意
全局异常过滤器部分支持 http-errors
库。基本上,任何包含 statusCode
和 message
属性的抛出异常都将被正确填充并作为响应发回(而不是用于无法识别的异常的默认 InternalServerErrorException
)。
抛出标准异常
Nest 提供了一个内置的 HttpException
类,从 @nestjs/common
包中暴露出来。对于典型的基于 HTTP REST/GraphQL API
的应用,最佳做法是在发生某些错误情况时发送标准 HTTP 响应对象。
例如,在 AppController
中,我们有一个 findAll()
方法(一个 GET 路由处理程序)。假设此路由处理程序出于某种原因抛出异常。为了证明这一点,我们将硬编码如下
@Get()
async findAll() {
throw new HttpException('Forbidden', HttpStatus.FORBIDDEN);
}
案例如下:
// app.controller.ts
import { Controller, Get, HttpException, HttpStatus } from '@nestjs/common';
import { AppService } from './app.service';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
async findAll() {
throw new HttpException('Forbidden', HttpStatus.FORBIDDEN);
}
}
访问路径:http://127.0.0.1:3000/
, 调用异常结果如下:
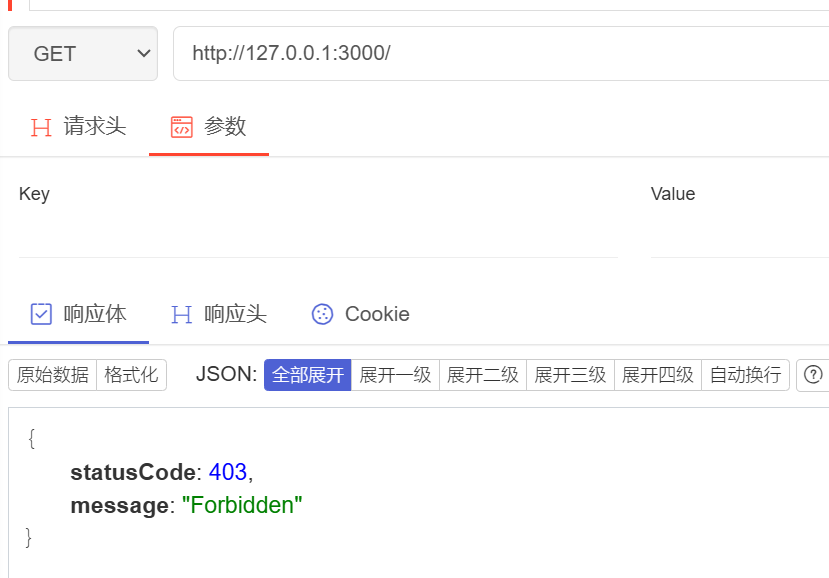
注意:这里使用的是 HttpStatus。这是从 @nestjs/common
包导入的辅助枚举。
当客户端调用此端点时,响应如下所示
{
"statusCode": 403,
"message": "Forbidden"
}
HttpException
构造函数采用两个必需的参数来确定响应:
response
参数定义 JSON 响应主体。它可以是string
或object
,如下所述。status
参数定义了 HTTP 状态代码。
默认情况下,JSON 响应主体包含两个属性:
statusCode
:默认为status
参数中提供的 HTTP 状态代码message
:基于status
的 HTTP 错误的简短描述
要仅覆盖 JSON 响应正文的消息部分,请在 response
参数中提供一个字符串。要覆盖整个 JSON 响应主体,请在 response
参数中传递一个对象。Nest 将序列化该对象并将其作为 JSON 响应主体返回。
第二个构造函数参数 - status
- 应该是有效的 HTTP 状态代码。最佳做法是使用从 @nestjs/common
导入的 HttpStatus
枚举。
有第三个构造函数参数(可选) - options
- 可用于提供错误 cause
。此 cause
对象未序列化到响应对象中,但它可用于记录目的,提供有关导致 HttpException
被抛出的内部错误的有价值信息。
这是一个覆盖整个响应主体并提供错误原因的示例
// app.controller.ts
@Get()
async findAll() {
try {
await this.service.findAll()
} catch (error) {
throw new HttpException({
status: HttpStatus.FORBIDDEN,
error: 'This is a custom message',
}, HttpStatus.FORBIDDEN, {
cause: error
});
}
}